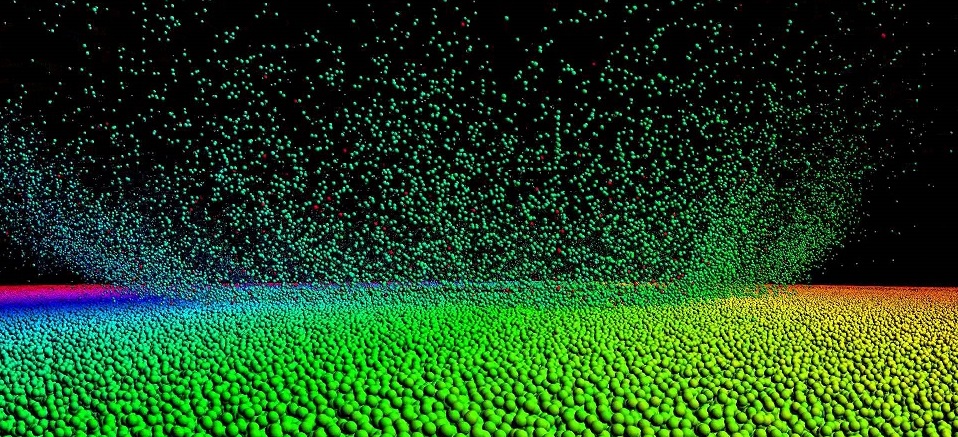
This weekend I decided to set up my own desktop to run CUDA programs for my GPU programming class. It probably wasn’t the best idea, since I had to force reboot my computer a few times for crashing my GeForce device driver. Oh well.
The approach I followed was from the Nvidia Getting Started Guide for Microsoft Windows. It’s pretty concise, so it’s definitely a good read if time allows.
How to Setup CUDA in Windows 10:
For this approach, you will need:
- A CUDA-enabled GPU
- Nvidia CUDA toolkit
- Visual Studio 2013
At the time of this writing (Nov 2015), Visual Studio 2014 and 2015 are not yet supported for CUDA programming. I believe Visual Studio is also free for non-commercial usage. I got my version free through Wake Forest University’s DreamSpark subscription.
To get started, download the latest CUDA toolkit from Nvidia’s official website and Visual Studio from Microsoft’s Visual Studio downloads page. The files are big, so you might want to leave it going for a while!
When Visual Studio finishes downloading, you will want to install that first (the toolkit recommends so). The VS file comes in an ISO format, so if you are on Windows 8 or 10, you can just right click the file to Mount the file. There will be no need for external ISO mounter programs.
If no windows pop up, head over to My Computer/This PC, and look under Devices and drives. The Visual Studio image will be there.
Once Visual Studio is installed, install the Nvidia CUDA toolkit. It’s also pretty intuitive, just click next next next and you’re done.
With both installed, let’s test the installation by opening up Visual Studio and selecting New Project.
If your CUDA toolkit installed successfully, there should be an option on the left column under Templates that says NVIDIA. Click onto NVIDIA, and select CUDA 7.5 (or whatever version you have installed). For name, let’s type in helloworld.
After clicking OK, kernel.cu should pop up in the editor. Copy in this very simple hello world program, and let’s run it.
#include <stdio.h> #include <device_launch_parameters.h> #include <cuda_runtime.h> #include <cuda.h> __global__ void add(int a, int b, int *c); int main() { int c; int *dev_c; cudaMalloc((void**)&dev_c, sizeof(int)); add <<<1, 1 >>>(2, 7, dev_c); cudaMemcpy(&c, dev_c, sizeof(int), cudaMemcpyDeviceToHost); printf("Hello world!\n"); printf("2 + 7 = %d\n", c); cudaFree(dev_c); exit(0); } __global__ void add(int a, int b, int *c) { c[0] = a + b; }
This simple program sends into the kernel as argument two integer values, and a pointer that points to the address of the allocated memory space in the GPU. In the kernel call, the two integers are added up, and the result is stored in the memory location that dev_c sent in. After the kernel call finishes, the data in the GPU memory will be copied back to the host, and stored into variable c.
We verify this operation by printing out the result.
To run the CUDA program, press CTRL + F5. In Visual Studio, the terminal terminates (pun intended, ha!) once the program finishes executing. With CTRL + F5, the terminal will stay open until you press any key to close it.
Another common way to circumvent this problem is to insert a cin statement at the end of main before the program terminates (if doing C++). Since the program will be waiting for an user input, the terminal will stay open until it receives something, or until you decide to kill it.
7 thoughts on “Setting up CUDA in Windows 10 (8, and 7 )”
Leave a Reply Cancel reply
This site uses Akismet to reduce spam. Learn how your comment data is processed.
I’d like to know why I can’t regsvr32 register the nvcuvenc.dll in system32 and syswow64 in windows 10… when it worked fine in 7…
I couldn’t get it to install on Windows 10, CUDA 7.5 installer just tells me that it doesn’t support this version of windows…
Any support?
I have a Windows 10 and it works fine. Try reinstalling CUDA?
I read in the documentation of the CUDA Toolkit that the installation may sometimes fail if a Windows update kicks in meanwhile. There could also be some other circumstances when the installation fails.
Reinstallation could help you
Thanks Kevin!
I used VS2013 Community with the newest CUDA toolkit on a Windows 10 and your guidelines work perfect!
Thank you!
Thanks you..Kevin.
I use CUDA 8.0, the program succeeded in build and run. But the output display for a second. It is mean that cuda with gpu run well. I am new in this field.
Hallo,
Can I also improve the performance of Adobe Premiere on a Win 10 platform, using this method! I am a complete dummy in this area!!!