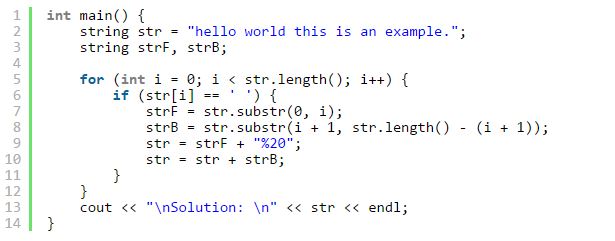
There are multiple ways to replace spaces in a string with “%20”, but one way I did it without starting from the back is through string concatenations and substrings (substr).
In C++:
int main() { string str = "hello world this is an example."; string strF, strB; for (int i = 0; i < str.length(); i++) { if (str[i] == ' ') { strF = str.substr(0, i); strB = str.substr(i + 1, str.length() - (i + 1)); str = strF + "%20"; str = str + strB; } } cout << "\nSolution: \n" << str << endl; }
Output:
hello%20world%20this%20is%20an%20example.
Substr takes in two inputs and returns a string that starts from position index and spans for length characters. In our above example, str.substr(0, i) when i = 5 ([5] == ‘ ‘) will return “hello” (starting from position 0 and spanning 5 chars).
My reasoning in this example was that I would take substrings of everything before and after the space, and then concatenate them together with a “%20” in between. Corner cases weren’t checked, but it works well in normal cases.
To check what were in the substrings, I inserted two cout statements — one after line 7 and one after line 8:
cout << "strF: " << strF << " "; strB = str.substr(i + 1, str.length() - (i + 1)); cout << "strB: " << strB << ". " << endl;
This gave an output of:
strF: hello strB: world this is an example.. strF: hello%20world strB: this is an example.. strF: hello%20world%20this strB: is an example.. strF: hello%20world%20this%20is strB: an example.. strF: hello%20world%20this%20is%20an strB: example.. Solution: hello%20world%20this%20is%20an%20example.